Introduction
This article shows you how we can create a User Control in WPF and how we can use it in our WPF applications. In this article, I'll show you how we can create a custom ToolTip in WPF with VS2008 SP1 & C#.
Background
There are similar articles like this, for example, see
this article that was written by Sacha Barber.
Using the Code
There we go. First we should create a User Control. Thus we have to select WPF User Control Library Project.
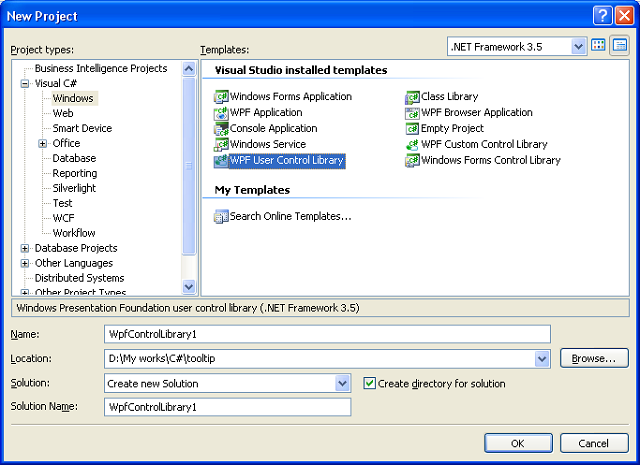
Now, we can create or edit the XAML code for creating a custom user control. I've used this XAML code for a custom tooltip. It's up to you what you want.
Collapse | Copy Code
<UserControl
Name="UserControlToolTip"
x:Class="CustomToolTip.UserControl1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d" RenderTransformOrigin="0,0" HorizontalAlignment="Left"
VerticalAlignment="Top" >
<UserControl.RenderTransform>
<TransformGroup>
<ScaleTransform ScaleX="1" ScaleY="1"/>
<SkewTransform AngleX="0" AngleY="0"/>
<RotateTransform Angle="0"/>
<TranslateTransform x:Name="UserControlToolTipXY" X="0" Y="0"/>
</TransformGroup>
</UserControl.RenderTransform>
<Grid HorizontalAlignment="Center" VerticalAlignment="Center"
MinWidth="200" MinHeight="120">
<Grid.RowDefinitions>
<RowDefinition Height="0.333*"/>
<RowDefinition Height="0.667*"/>
</Grid.RowDefinitions>
<Rectangle Fill="#FFFBFBFB" Stroke="#FF000000" RadiusX="10" RadiusY="10"
RenderTransformOrigin="0.139,0.012" StrokeThickness="1" Grid.RowSpan="2">
<Rectangle.BitmapEffect>
<DropShadowBitmapEffect Opacity="0.8"/>
</Rectangle.BitmapEffect>
</Rectangle>
<Rectangle RadiusX="10" RadiusY="10" RenderTransformOrigin="0.139,0.012"
StrokeThickness="10" Stroke="{x:Null}"
Margin="1,1,1,1" Grid.Row="0" Grid.RowSpan="2">
<Rectangle.Fill>
<LinearGradientBrush EndPoint="0.5,1" StartPoint="0.5,0.725">
<GradientStop Color="#00E6D9AA" Offset="0.487"/>
<GradientStop Color="#FFE6D9AA" Offset="0.996"/>
</LinearGradientBrush>
</Rectangle.Fill>
</Rectangle>
<Rectangle RadiusX="10" RadiusY="10" RenderTransformOrigin="0.493,0.485"
StrokeThickness="10" Stroke="{x:Null}" Grid.RowSpan="2" Margin="1,1,1,1">
<Rectangle.Fill>
<LinearGradientBrush EndPoint="0.014,0.5" StartPoint="0.211,0.5">
<GradientStop Color="#00E6D9AA" Offset="0.513"/>
<GradientStop Color="#FFE6D9AA" Offset="0.996"/>
</LinearGradientBrush>
</Rectangle.Fill>
</Rectangle>
<Rectangle RadiusX="10" RadiusY="10" RenderTransformOrigin="0.493,0.485"
StrokeThickness="10" Stroke="{x:Null}" Grid.RowSpan="2" Margin="1,1,1,1">
<Rectangle.Fill>
<LinearGradientBrush EndPoint="0.493,0.002" StartPoint="0.493,0.33">
<GradientStop Color="#00E6D9AA" Offset="0.513"/>
<GradientStop Color="#FFE6D9AA" Offset="0.996"/>
</LinearGradientBrush>
</Rectangle.Fill>
</Rectangle>
<Rectangle RadiusX="10" RadiusY="10" RenderTransformOrigin="0.493,0.485"
StrokeThickness="10" Stroke="{x:Null}" Grid.RowSpan="2" Margin="1,1,1,1">
<Rectangle.Fill>
<LinearGradientBrush EndPoint="0.99,0.441" StartPoint="0.794,0.441">
<GradientStop Color="#00E6D9AA" Offset="0.513"/>
<GradientStop Color="#FFE6D9AA" Offset="0.996"/>
</LinearGradientBrush>
</Rectangle.Fill>
</Rectangle>
<TextBlock Text="TextBlock" TextWrapping="Wrap" x:Name="TextBlockToolTip"
RenderTransformOrigin="0.5,0.5" Grid.Row="1" HorizontalAlignment="Left"
VerticalAlignment="Center" Margin="20,0,0,20" />
<TextBlock Name="ToolTipTitle" HorizontalAlignment="Stretch" Margin="15,16,15,6.1"
FontSize="14" Text="title" d:LayoutOverrides="Height" />
</Grid>
</UserControl>
Also, I've added these methods and properties for controlling the elements:
Collapse | Copy Code
namespace CustomToolTip
{
public partial class UserControl1 : UserControl
{
public UserControl1()
{
InitializeComponent();
}
public double UserControlToolTipX
{
get { return this.UserControlToolTipXY.X; }
set { this.UserControlToolTipXY.X = value; }
}
public double UserControlToolTipY
{
get { return this.UserControlToolTipXY.Y; }
set { this.UserControlToolTipXY.Y = value; }
}
public string UserControlTextBlockToolTip
{
get { return TextBlockToolTip.Text; }
set { TextBlockToolTip.Text = value; }
}
public string UserControlToolTipTitle
{
get { return ToolTipTitle.Text; }
set { ToolTipTitle.Text = value; }
}
}
}
After that, press Shift + F6 for building the DLL file. Now we can create a WPF application and use our custom user control in it. Thus, we should choose a WPF Application Project.

Then, we have to add our User Control DLL file in the references.
We are going to use the custom User Control within a XAML window. So we have to add an extra XAML code. We have to add the following line within Window
element:
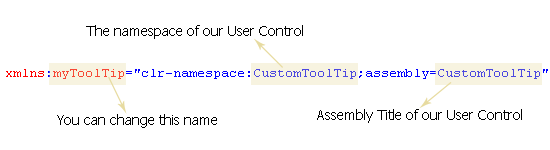
At last, we must have a Window tag in our XAML code like this:
Collapse | Copy Code
<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:myToolTip="clr-namespace:CustomToolTip;assembly=CustomToolTip"
Title="Window1" Height="600" Width="800">
</Window>
OK, now we can use the User Control in the XAML code with XAML code like this:
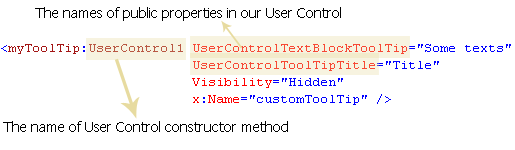
At last, I've created this XAML code:
Collapse | Copy Code
<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:myToolTip="clr-namespace:CustomToolTip;assembly=CustomToolTip"
Title="Window1" Height="600" Width="800">
<Grid x:Name="rootGrid" RenderTransformOrigin="0.5,0.5">
<Grid.RenderTransform>
<TransformGroup>
<ScaleTransform ScaleX="1" ScaleY="1"/>
<SkewTransform AngleX="0" AngleY="0"/>
<RotateTransform Angle="0"/>
<TranslateTransform x:Name="rootGridXY" X="0" Y="0"/>
</TransformGroup>
</Grid.RenderTransform>
<Rectangle Margin="26,34,496,374" Name="rectangle1" Stroke="Black"
Fill="Coral" MouseLeave="rectangle_MouseLeave"
MouseMove="rectangle_MouseMove" />
<Rectangle Fill="Lavender" Margin="537,29,53,376" Name="rectangle2"
Stroke="Black" MouseMove="rectangle_MouseMove"
MouseLeave="rectangle_MouseLeave" />
<Rectangle Fill="Peru" Margin="192,391,186,37.995" Name="rectangle3"
Stroke="Black" MouseMove="rectangle_MouseMove"
MouseLeave="rectangle_MouseLeave" />
<myToolTip:UserControl1 UserControlTextBlockToolTip="Some texts"
UserControlToolTipTitle="Title"
Visibility="Hidden"
x:Name="customToolTip" />
</Grid>
</Window>
And these are the methods for showing or hiding our custom ToolTip:
Collapse | Copy Code
namespace WpfApplication1
{
public partial class Window1 : Window
{
public Window1()
{
InitializeComponent();
}
private void rectangle_MouseLeave(object sender, MouseEventArgs e)
{
state = true;
customToolTip.Visibility = Visibility.Hidden;
}
bool state = true;
Random rand = new Random(DateTime.Now.Millisecond);
private void rectangle_MouseMove(object sender, MouseEventArgs e)
{
if (state)
{
customToolTip.Visibility = Visibility.Visible;
customToolTip.UserControlToolTipTitle =
(sender as Rectangle).Name.ToUpperInvariant();
customToolTip.UserControlTextBlockToolTip = "";
for (int i = 0; i < rand.Next(1, 30); i++)
customToolTip.UserControlTextBlockToolTip += (sender as Rectangle).Name
+ "\t" + i.ToString() + "\n";
}
customToolTip.UserControlToolTipX = Mouse.GetPosition(this).X + 10;
customToolTip.UserControlToolTipY = Mouse.GetPosition(this).Y + 10;
state = false;
}
}
}
That's all.