Variables
In our school life we learned about variables and equations in algebra. Let assume a=30, b=12 and c= a-b, then c=18, i.e. variables like a, b, c store numbers. In JavaScript and other programming language a variable contain values (piece of information) and stores in computer's memory which is changeable.
Naming variables
There are five rules for naming variables in JavaScript.
- The first character must be a letter (a-z, A-Z) or an underscore (_).
- The rest of the name can be made up of letters (a-z, A-Z), numbers (0-9) or underscore(_).
- Variable name cannot contains space character.
- In JavaScript variables are case sensitive, so emp_code is different from Emp_Code.
- We should not use the "reserve words" like alert, var as variable name. See the list of Reserve Words.
Pictorial presentation of JavaScript variable naming
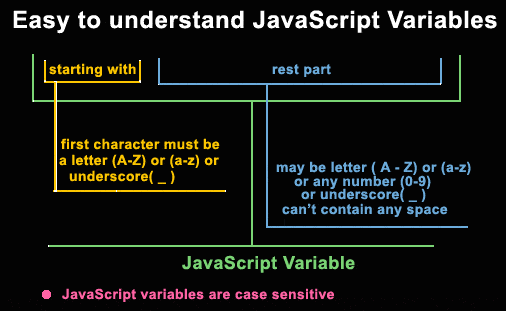
Invalid Variable Name
- var // var is reserve word.
- 77employee // Initial character is a number.
- Variable%name // % character is not allowed.
- Name&code // & is not allowed.
Valid Variable Name
- employee_77
- Variable_name
- x
- Name_code
- name_code
- _emp_name
Declaring Variables
We can declare a variable in the following way :
With the keyword var, followed by name of the variable. For example, to declare a new variable called emp_name, the statement is -
With the keyword var, followed by name of the variable. For example, to declare a new variable called emp_name, the statement is -
var emp_name
The above syntax can be used to declare both local and global variables.
Another way to declare a variable is simply assigning it a value. For example
- x=12.2
- emp_code = 'E123'
Therefore var x = 12.5 and x = 12.5 are equal.
// Declaring a variable
// Best Practices
var emp_code = 'E123';
// Poor Practices
emp_code = 'E123'
// Best Practices
var emp_code = 'E123';
// Poor Practices
emp_code = 'E123'
// Declaring multiple variables. Use one var statement and declare each variable on a new line.
// Best Practices
var emp_list = emplist(),
x = 12,
str1 = 'String1' ;
// Poor Practices
var emp_code = emplist();
var x = 12 ;
var str1 = 'String1';
// Best Practices
var emp_list = emplist(),
x = 12,
str1 = 'String1' ;
// Poor Practices
var emp_code = emplist();
var x = 12 ;
var str1 = 'String1';
Evaluating Variables
A variable or array element that has not been assigned a value, has the value undefined. The result of evaluating an unassigned variable depends on how it was declared:
If the unassigned variable was declared with var, the evaluation results in the undefined value or NaN in numeric contexts.
If the unassigned variable is declared without var statement, the evaluation results in a run time error.
See the following statements :
- var x;
- print("The value of x is : " + x); // prints "The value of x is : undefined"
- print("The value of y is : " + y); // throws reference error exception
You can use undefined to determine whether a variable has a value.
In the following statements, the variable x is not assigned a value, and the if statement evaluates to true.
- var x;
- if(x === undefined)
- {
- do something ;
- }
- else
- {
- do something ;
- }
Variables Usage
The variables can be declared and used in two ways locally and globally. When you declare a JavaScript variable within a function, it is called a local variable because the variable is available only within the function. You can use same variable name within different function. When you declare a variable outside a function it is called global variable.
You can optionally declare a global variable using var (for example var empCode). However, you must use var to declare a variable inside a function.
JavaScript : Constants
In JavaScript, constants are declared with const keyword and assigned at the time of the declaration. A constant can be global or local to a function where it is declared
Constants are read-only, therefore you can not modify them later on.
Naming a constant in JavaScript follow the same rule of naming a variable except that the const keyword is always required, even for global constants.
If the keyword is omitted, the identifier is assumed to represent a variable.
Rules for naming constants in JavaScript
- The first character must be a letter (a-z, A-Z) or an underscore (_).
- The rest of the name can be made up of letters (a-z, A-Z), numbers (0-9) or underscore(_).
- Name of constant can not contains space character.
- In JavaScript, constants are case sensitive, so emp_code is different from Emp_Code.
- We should not use the the "reserve words" like alert, var as constants name. See the list of Reserve Words.
Pictorial presentation of JavaScript constant naming
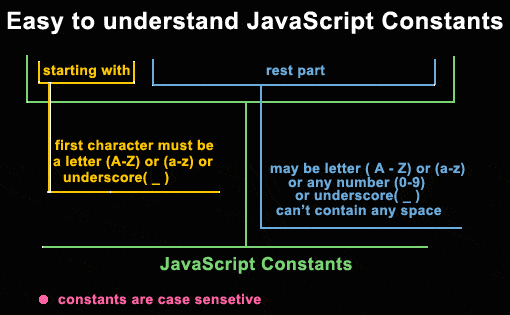
Example
- const country = 'India';
- //You cannot declare a constant with the same name as a function
- // or variable in the same scope. Following statements create error.
- function abc()
- {
- const abc = 55;
- }
- function abc()
- {
- const x = 15;
- var x;
- }
JavaScript : Data Type
A value is a piece of information that can be a Number, String, Boolean, Null etc. JavaScript recognizes the following types of values.
Types | Description | Example |
---|---|---|
String | A series of characters enclosed in quotation marks. A string must be delimited by quotation marks of the same type, either single quotation marks ( ') or double quotation marks ("). | "google.com", 'yahoo.com' |
Numbers | Any numeric value. The numbers can be either positive or negative. | 45, 45887, -45, 12.23, -145.55 |
Logical (Boolean) | A logical true or false. Use to evaluate whether a condition is true or false. | true, false |
null | A special keyword denoting a null value (i.e. empty value or nothing). Since JavaScript is case-sensitive, null is not the same as Null, NULL, or any other variant. | |
undefined | A top-level property whose value is undefined, undefined is also a primitive value. |
JavaScript : Data Type Conversion
JavaScript is a dynamically typed language. Therefore there is no need to specify the data type of a variable at the time of declaring it. Data types are converted automatically as needed during script execution.
For example, we define the age of a person in the 'age' variable as follows :
- var age = 21
Let store a string value to the said variable.
- age = "What is your age?"
As JavaScript is dynamically typed, the said assignment does not create any error.
JavaScript : Expressions
The following expressions contain string values, numeric values and + operator. JavaScript converts numeric values to strings.
- x = "What is your age ?" + 21 // returns "What is your age ?21 "
- y = 21 + " is my age. " // returns "21 is my age."
The following expressions contain + and - operators.
- "21" - 7 // returns 14
- "21" + 7 // returns "217"
- "21" + "7" // returns "217"
The following expressions contain * and / operators.
- "21" * 7 // returns 147
- "21" / 7 // returns 3
JavaScript : Reserved Words
The words in the following table are reserved words and cannot be used as JavaScript variables, functions, methods, or object names. Some of these words are keywords, used in JavaScript ; others are reserved for future use.
List of reserved words
break | for | throw |
case | function | try |
catch | if | typeof |
continue | in | var |
default | instanceof | void |
delete | new | while |
do | return | with |
else | switch | |
finally | this |
The following words are reserved as future keywords
abstract | export | long | synchronized |
boolean | extends | native | throws |
byte | final | package | transient |
char | float | private | volatile |
class | goto | protected | |
const | implements | public | |
debugger | import | short | |
double | int | static | |
enum | interface | super |
great
ReplyDelete